GA의 원하는 데이터와 쇼핑몰 매출데이터를 한번에 보기위해 어떤 방법이 있을까 고민하던중 GA API를 사용하어 데이터를 가져오기로 했다. 구글에서 작성한 튜토리얼 문서가 있어 이 문서를 참조하여 진행한다. 최근 GA 버전이 업데이트 되면서 v4 문서도 있으니 상황에 맞게 사용하면 된다. v3 가이드 문서로 진행한다.
developers.google.com/analytics/devguides/config/mgmt/v3/quickstart/service-py
Hello Analytics API: Python quickstart for service accounts
This tutorial walks through the steps required to access a Google Analytics account, query the Analytics APIs, handle the API responses, and output the results. The Core Reporting API v3.0, Management API v3.0, and OAuth2.0 are used in this tutorial. Note:
developers.google.com
GA API 활성화
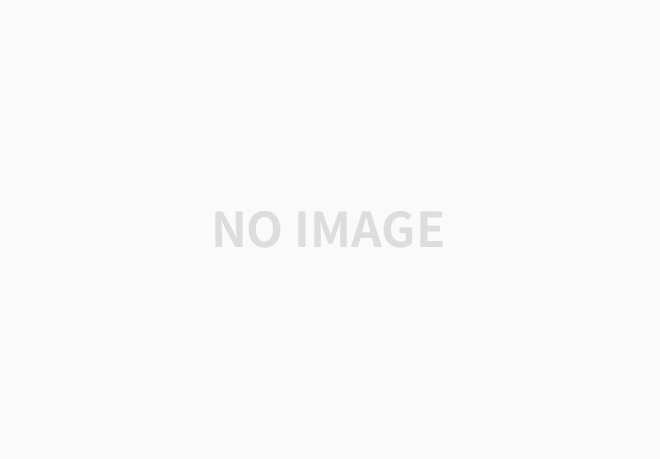
문서의 링크를 클릭하여 개발자 콘솔에서 GA API를 활성화한다.
사용중인 프로젝트를 선택하거나 새로운 프로젝트를 생성한다. 기존의 프로젝트를 사용했다.
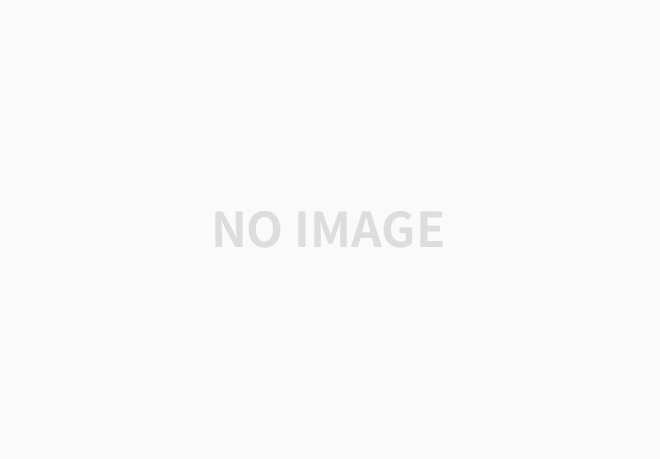
사용자 인증 정보를 등록한다.
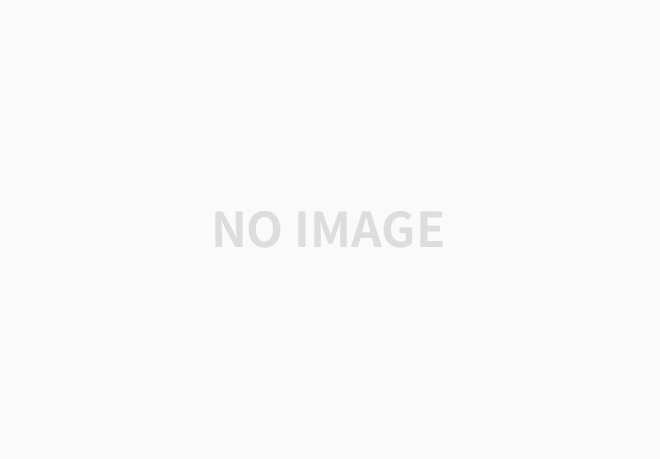
API사용할 환경을 선택하고 어떤 데이터를 접근할지 선택한다. 선택 후 어떤 사용자 인증 정보가 필요한가요? 버튼을 클릭하면 인증정보를 확인 할 수 있다.
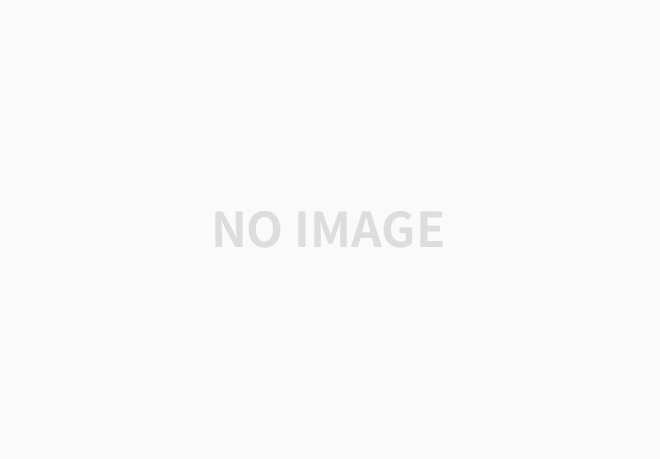
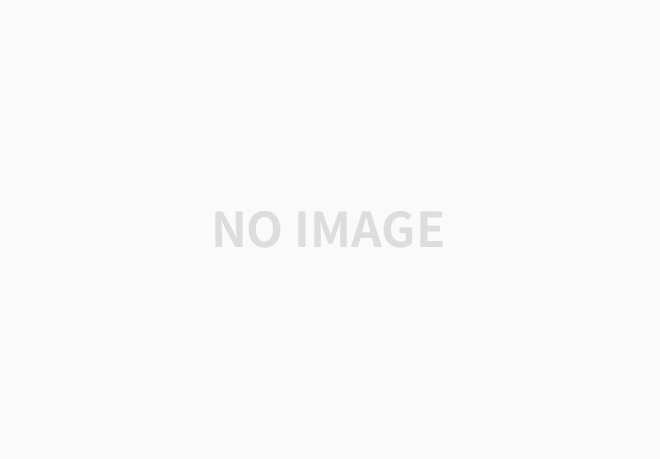
서비스 계정 추가
console.developers.google.com/iam-admin/serviceaccounts 이동하여 서비스 계정을 추가하도록 한다. 먼저 사용할 프로젝트를 선택
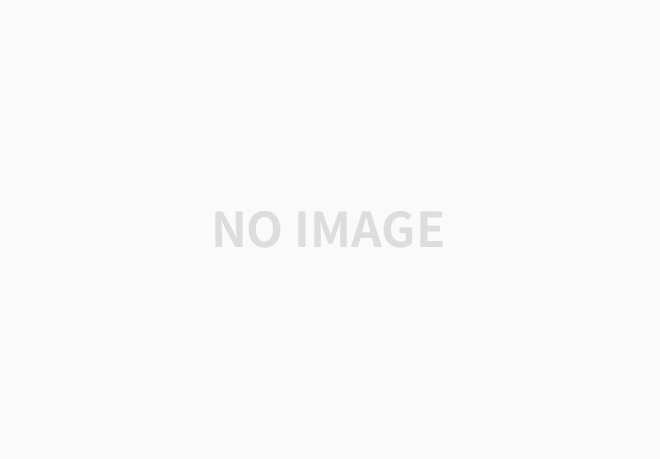
프로젝트 선택후 상단의 서비스 계정 만들기를 클릭한다.
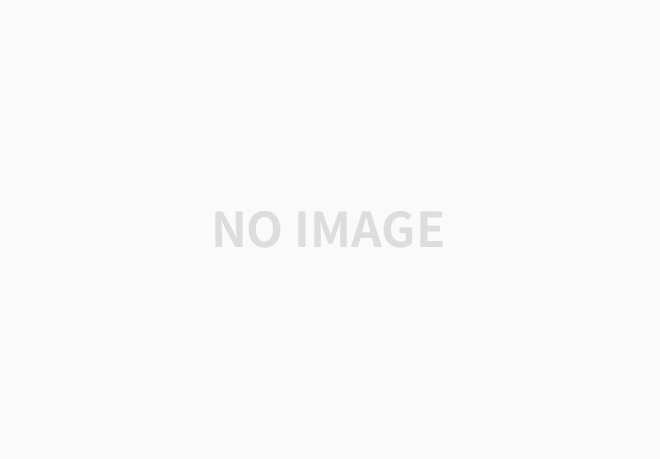
계정 이름을 입력하면 id는 랜덤으로 생성해준다.
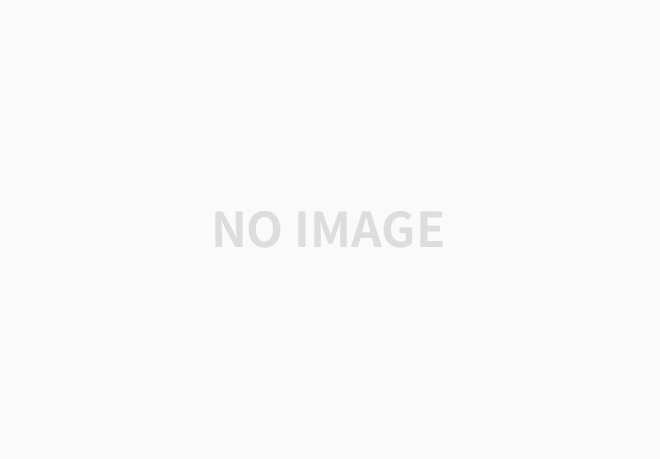
권한 부여 단계와 액세스 권한 부여 단계는 건너 뛴다. 계속을 클릭.
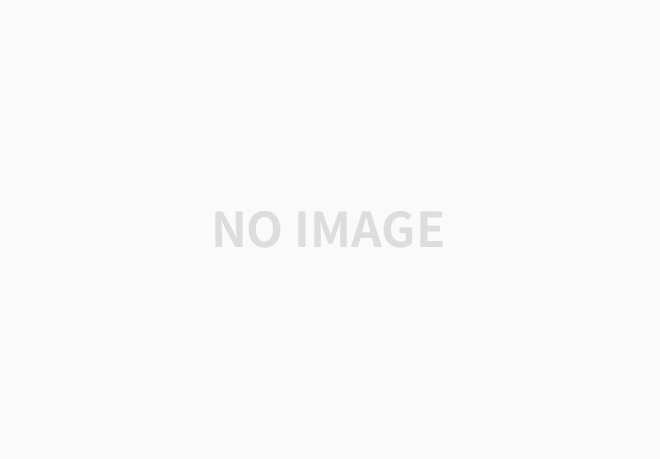
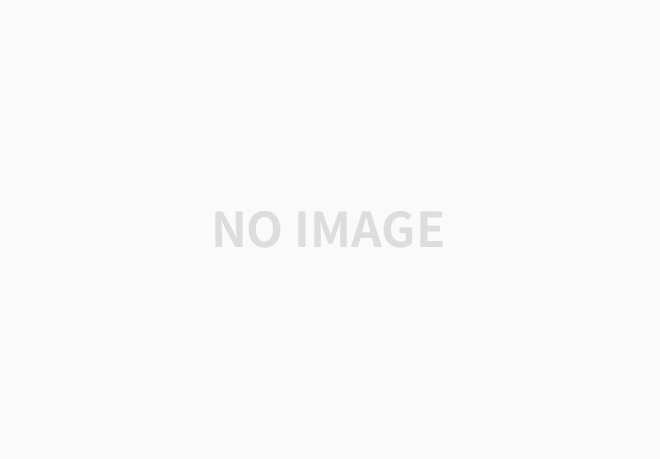
계정이 추가되었다. 계정 이메일을 클릭하여 설정을 진행한다.
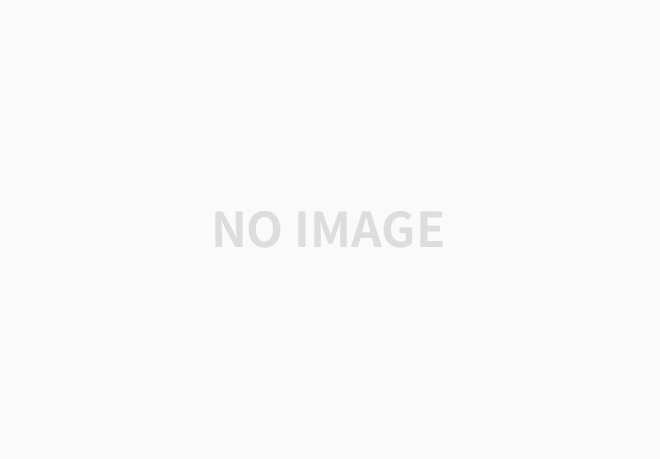
하단에서 키 추가를 클릭하면 json파일이 다운로드 된다.
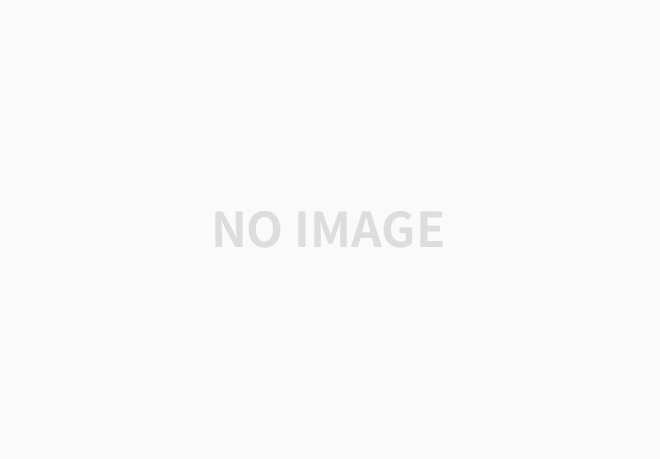
GA 서비스 계정 등록
GA 설정 메뉴에서 서비스 계정을 등록하여 API를 설정을 마무리 한다.
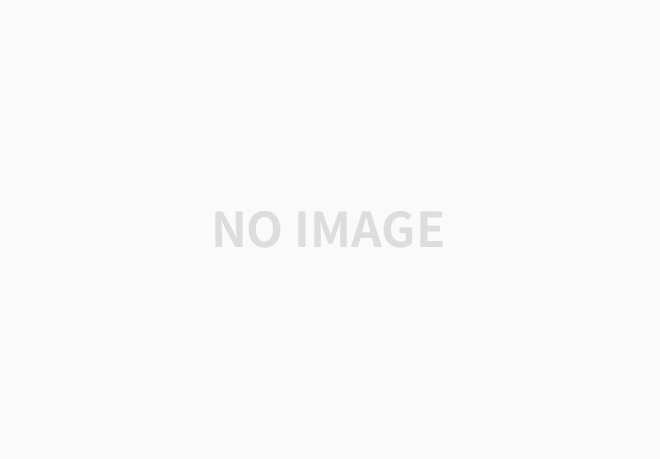
생성된 사용자 계정을 추가한다.
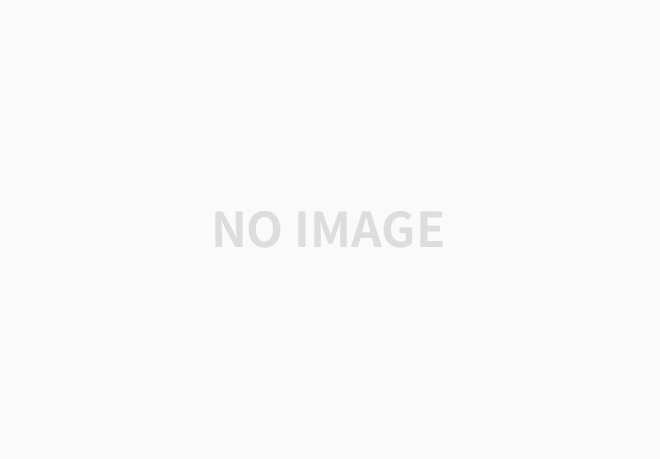
파이썬 코드 작성
구글 api 패키지를 설치한다.
sudo pip install --upgrade google-api-python-client
가이드 문서의 참조하여 api 코드를 하나하나 살펴본다. 계정 정보와 속성 정보를 받아오는 부분이다.
from apiclient.discovery import build
from oauth2client.service_account import ServiceAccountCredentials
from pprint import pprint
def get_service(api_name, api_version, scopes, key_file_location):
"""Get a service that communicates to a Google API.
Args:
api_name: The name of the api to connect to.
api_version: The api version to connect to.
scopes: A list auth scopes to authorize for the application.
key_file_location: The path to a valid service account JSON key file.
Returns:
A service that is connected to the specified API.
"""
credentials = ServiceAccountCredentials.from_json_keyfile_name(
key_file_location, scopes=scopes)
# Build the service object.
service = build(api_name, api_version, credentials=credentials)
return service
# Define the auth scopes to request.
scope = 'https://www.googleapis.com/auth/analytics.readonly'
key_file_location = '/Users/dooyeoung/Documents/googleapi/instanttattoo-273a7a5b7269_ga.json'
# Authenticate and construct service.
service = get_service(
api_name='analytics',
api_version='v3',
scopes=[scope],
key_file_location=key_file_location)
# Get a list of all Google Analytics accounts for this user
accounts = service.management().accounts().list().execute()
if accounts.get('items'):
# Get the first Google Analytics account.
account = accounts.get('items')[0].get('id')
# Get a list of all the properties for the first account.
properties = service.management().webproperties().list(accountId=account).execute()
# 일주일간 세션수와, 페이지뷰수 받아오기
result = service.data().ga().get(
ids='ga:191315342',
start_date='7daysAgo',
end_date='today',
metrics='ga:sessions,ga:pageviews').execute()
accounts 변수에 ga 액세스 계정 정보있고 properties에 속성 정보가 있다.
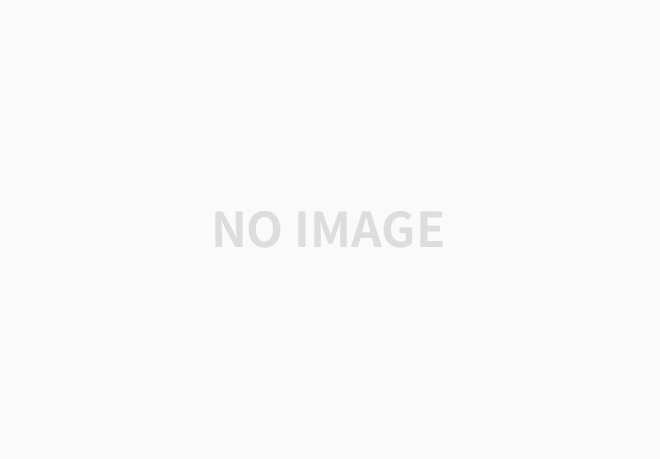
result 변수에 쿼리 결과값은 아래와 같다. columnHeaders와 rows 부분만 필요하기에 추출하여 사용하면 된다.
{
"kind": "analytics#gaData",
"id": "https://www.googleapis.com/analytics/v3/data/ga?ids=ga:191315342&metrics=ga:sessions,ga:pageviews&start-date=7daysAgo&end-date=today",
"query": {
"start-date": "7daysAgo",
"end-date": "today",
"ids": "ga:191315342",
"metrics": [
"ga:sessions",
"ga:pageviews"
],
"start-index": 1,
"max-results": 1000
},
"itemsPerPage": 1000,
"totalResults": 1,
"selfLink": "https://www.googleapis.com/analytics/v3/data/ga?ids=ga:191315342&metrics=ga:sessions,ga:pageviews&start-date=7daysAgo&end-date=today",
"profileInfo": {
"profileId": "191315342",
"accountId": "136003283",
"webPropertyId": "UA-136003283-2",
"internalWebPropertyId": "196231664",
"profileName": "All Web Site Data",
"tableId": "ga:191315342"
},
"containsSampledData": false,
"columnHeaders": [
{
"name": "ga:sessions",
"columnType": "METRIC",
"dataType": "INTEGER"
},
{
"name": "ga:pageviews",
"columnType": "METRIC",
"dataType": "INTEGER"
}
],
"totalsForAllResults": {
"ga:sessions": "16101",
"ga:pageviews": "44265"
},
"rows": [
[
"16101",
"44265"
]
]
}
코드 작성 전 query explorer를 참조하면 좀 더 쉽게 코드 작성이 가능하니 적극 활용 하기로 한다.
ga-dev-tools.appspot.com/query-explorer/
Query Explorer — Google Analytics Demos & Tools
Overview Sometimes you just need to explore. This tool lets you play with the Core Reporting API by building queries to get data from your Google Analytics views (profiles). You can use these queries in any of the client libraries to build your own tools.
ga-dev-tools.appspot.com
일주일동안 페이지별 순 페이지 뷰수를 내림차순으로 정렬하여 데이터를 가져오려한다. query selector를 사용하여 아래와 같이 결과 예시를 볼 수 있다.
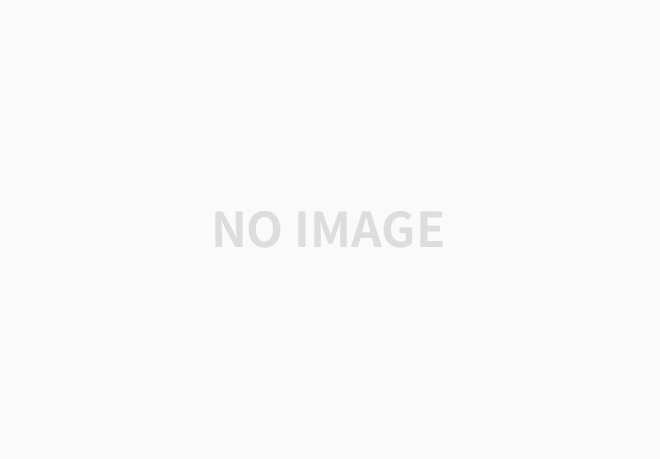
위 내용을 python code로 옮기면 아래와 같다.
result = service.data().ga().get(
ids='ga:191315342',
start_date='7daysAgo',
end_date='today',
metrics='ga:uniquePageviews',
dimensions='ga:pagePath',
sort="-ga:uniquePageviews").execute()
rdf = pd.DataFrame(result['rows'],
columns=[col['name'] for col in result['columnHeaders']] )
rdf.head(10)
api 문서에서 파리미터 설명과 사용법을 참고하도록 한다.
developers.google.com/analytics/devguides/reporting/core/v3/reference#ids
Core Reporting API - Reference Guide | Analytics Core Reporting API
itemsPerPage integer The maximum number of rows the response can contain, regardless of the actual number of rows returned. If the max-results query parameter is specified, the value of itemsPerPage is the smaller of max-results or 10,000. The default valu
developers.google.com
'Python' 카테고리의 다른 글
Python - Django REST Framework 초기 설정 (0) | 2020.12.29 |
---|---|
python - 페이스북,인스타그램 광고데이터 수집 자동화 (3) | 2020.12.22 |
python - cafe24 매출 데이터 수집 자동화 (0) | 2020.12.18 |